🧰 Minimal Nuxt 3 starter with Vuetify, Pinia and Google login
I believe you have already read my 👉 Nuxt 3 migration guide 👈.
And in this blog, I’ll be covering exactly what was mentioned there
— an example demo project that includes Vue 3, Nuxt 3, Vuetify 3, Pinia, PWA, and Firebase authentication.
Find the source code here, making it an excellent starter for those new to Nuxt 3.
The motivation behind creating this starter was rooted in the complexity of the project I was migrating. It became challenging to pinpoint certain errors, making the debugging process cumbersome. Thus, having a minimal demo project like this proved to be invaluable, as it allows for swift code validation and configuration testing. With this straightforward starter, you can focus on honing your skills and building your Nuxt 3 applications with confidence.
🚛 Sure, let me walk you through how I built up this starter:
- Initializing a Nuxt 3 Project
- Adding TypeScript Configuration
- Adding ESLint and lint-staged
- Adding Internationalization (i18n)
- Adding Vuetify
- Utilizing SCSS Variables
- Adding Pinia
- Adding Firebase Google Login
Step 1: Initializing a Nuxt 3 Project
To kickstart our journey with Nuxt 3, the first step is to create a new project. Keep in mind that Nuxt 3 requires Node.js version 16.10.0 or newer, so ensure you have the appropriate version installed on your machine.
To create the project, open your terminal and run the following command, replace <project-name>
with the desired name for your project. This command will initialize a new Nuxt 3 project in the specified folder:
1 | npx create-nuxt-app <project-name> |
Next, navigate to the project folder and then run:
1 | cd <project-name> |
It’s time to install the necessary dependencies. With everything set up, the next command allowed to run the Nuxt 3 project locally and start developing:
1 | yarn install |
Step 2: Adding TypeScript Configuration
To further enhance our Nuxt 3 project and leverage the benefits of TypeScript, let’s integrate TypeScript configuration. TypeScript brings static type checking, improved code readability, and better developer tooling, making it a valuable addition to the project.
First, install TypeScript using Yarn:
1 | yarn add --dev typescript |
Next, create a tsconfig.json
file in the root of the project. This file serves as the configuration file for TypeScript, enabling us to customize its behavior according to the project’s needs. Here’s an example:
1 | // tsconfig.json |
Step 3: Adding ESLint and lint-staged
To maintain a consistent and error-free codebase, I implemented ESLint and lint-staged in our Nuxt 3 project. ESLint provides static code analysis to identify and fix code errors, while lint-staged allows us to run ESLint on staged files, ensuring that only the committed code meets our coding standards.
To begin, install ESLint and its associated dependencies using Yarn:
1 | yarn add --dev eslint eslint-plugin-nuxt lint-staged |
Next, create an .eslintrc.js
file in the root of the project. This file contains the ESLint configuration, including any rules and plugins we want to enforce. I customized the configuration to suit our project’s needs, considering both Vue.js and TypeScript specifics.
1 | // .eslintrc |
In the package.json
file, add the ESLint scripts and configuring lint-staged:
- lint:js: This script runs ESLint to check all JavaScript, TypeScript, and Vue files in the project, excluding the ones specified in the .gitignore file.
- lint: This is an alias for yarn lint:js, which means you can now use yarn lint to execute the ESLint checks.
- lintfix: This script also runs ESLint, but with the –fix flag, which automatically attempts to fix any fixable issues in the code.
- lint-staged: This configuration specifies that lint-staged should run ESLint with the –fix flag only on TypeScript (.ts) and Vue (.vue) files that are staged for a commit.
1 | // package.json |
Step 4: Adding Internationalization (i18n)
To cater to a global audience and provide a localized experience, I introduced internationalization (i18n) to our Nuxt 3 project. i18n enables us to translate the content and messages in our application into multiple languages, enhancing accessibility and user engagement.
First, install @nuxtjs/i18n
using Yarn:
1 | yarn add --dev @nuxtjs/i18n |
Then, add @nuxtjs/i18n
to the modules section in your nuxt.config. You can use either of the following ways to specify the module options:
1 | export default defineNuxtConfig({ |
Next, create an i18n.config.ts
file to set up the i18n configuration. In this file, I defined the supported languages, default language, and any other relevant settings for translation.
1 | export default { |
To allow users to switch between different languages, I added a language switcher to the user interface. This component lets users select their preferred language and updates the content accordingly.
1 | <template> |
Step 5: Adding Vuetify
To enrich the user interface and provide a cohesive design language, I integrated Vuetify into our Nuxt 3 project. Vuetify offers a comprehensive set of pre-designed components and styles, enabling us to create visually stunning and responsive user interfaces with minimal effort.
First, install vuetify
using Yarn:
1 | yarn add --dev vuetify |
Next, add the necessary Vuetify configuration to the nuxt.config.js
file.
1 | build: { transpile: ['vuetify'] }, |
Then, create a plugin named vuetify.ts
under plugins folder, ensures that Vuetify is properly loaded and available across the entire application.
1 | import { defineNuxtPlugin } from 'nuxt/app' |
Vuetify comes with two themes pre-installed, light and dark. Now let’s add Vuetify theme toggle to validate if it can work.
1 | <template> |
Step 6: Utilizing SCSS Variables
To leverage the power of SCSS variables in our Nuxt 3 project and enhance code reusability and maintainability, I introduced SCSS variables. SCSS variables allow us to store commonly used values, such as colors or font sizes, in one place and use them across our stylesheets.
In the Vuetify configuration within the plugins/vuetify.ts
file, I used SCSS variables to define custom theme colors.
1 | // plugins/vuetify.ts |
In the styles.scss
file, I defined the SCSS variables $my-color1
and $my-color2
, representing colors used in the application. By using SCSS variables, we can easily change these colors in one place and see the changes propagate throughout the project.
1 | // styles.scss |
1 | <template> |
Step 7: Adding Pinia
To enhance state management and improve the overall architecture of our Nuxt 3 project, I integrated Pinia. Pinia is a modern, Vue.js-based state management solution that offers simplicity, reactivity, and excellent TypeScript support.
First, install TypeScript using Yarn:
1 | yarn add pinia @pinia/nuxt |
Then add it to modules in nuxt.config.js
file:
1 | // nuxt.config.js |
By default @pinia/nuxt
exposes one single auto import: usePinia()
, you can add auto imports for other Pinia related utilities like this:
1 | // nuxt.config.js |
Here’s a simple example defining a store using pinia in Option API.
1 | export const useCounterStore = defineStore('counter', { |
1 | <template> |
Step 8: Adding Firebase Google Login
To enable users to log in to our Nuxt 3 application using their Google accounts, we can integrate Firebase Authentication. Firebase Authentication offers a secure and straightforward way to authenticate users and manage their login sessions.
First, install firebase dependencies:
1 | yarn add firebase firebase-admin |
Create a new file named firebase.client.ts inside the plugins folder. In this file, initialize Firebase for the client-side of our Nuxt 3 application:
1 | // plugins/firebase.client.ts |
For server-side rendering (SSR), you may need a separate Firebase configuration file named firebase.server.ts inside the plugins folder. However, since Firebase Authentication works on the client-side, you may not need this file for our current implementation.
1 | // plugins/firebase.server.ts |
Create a new file named useAuth.ts inside the composables folder. This composable will handle Firebase Authentication and provide methods for signing in with Google, signing out, and accessing the user’s information:
1 | // composables/useAuth.ts |
Now, create a new Vue component named Login.vue in the appropriate folder. This component will display a “Sign In with Google” button when the user is not logged in and a “Welcome back” message with a “Sign Out” button when the user is logged in:
1 | <template> |
With these components and configurations in place, our Nuxt 3 project is now equipped with Firebase Google Login. Users can easily sign in using their Google accounts, and once authenticated, the application can display personalized content and features based on the user’s login status.
Feel free to customize and expand upon this blog post to include additional features and considerations specific to your project. Happy writing and best of luck with your Nuxt 3 application!
Find the source code here, making it an excellent starter for those new to Nuxt 3.
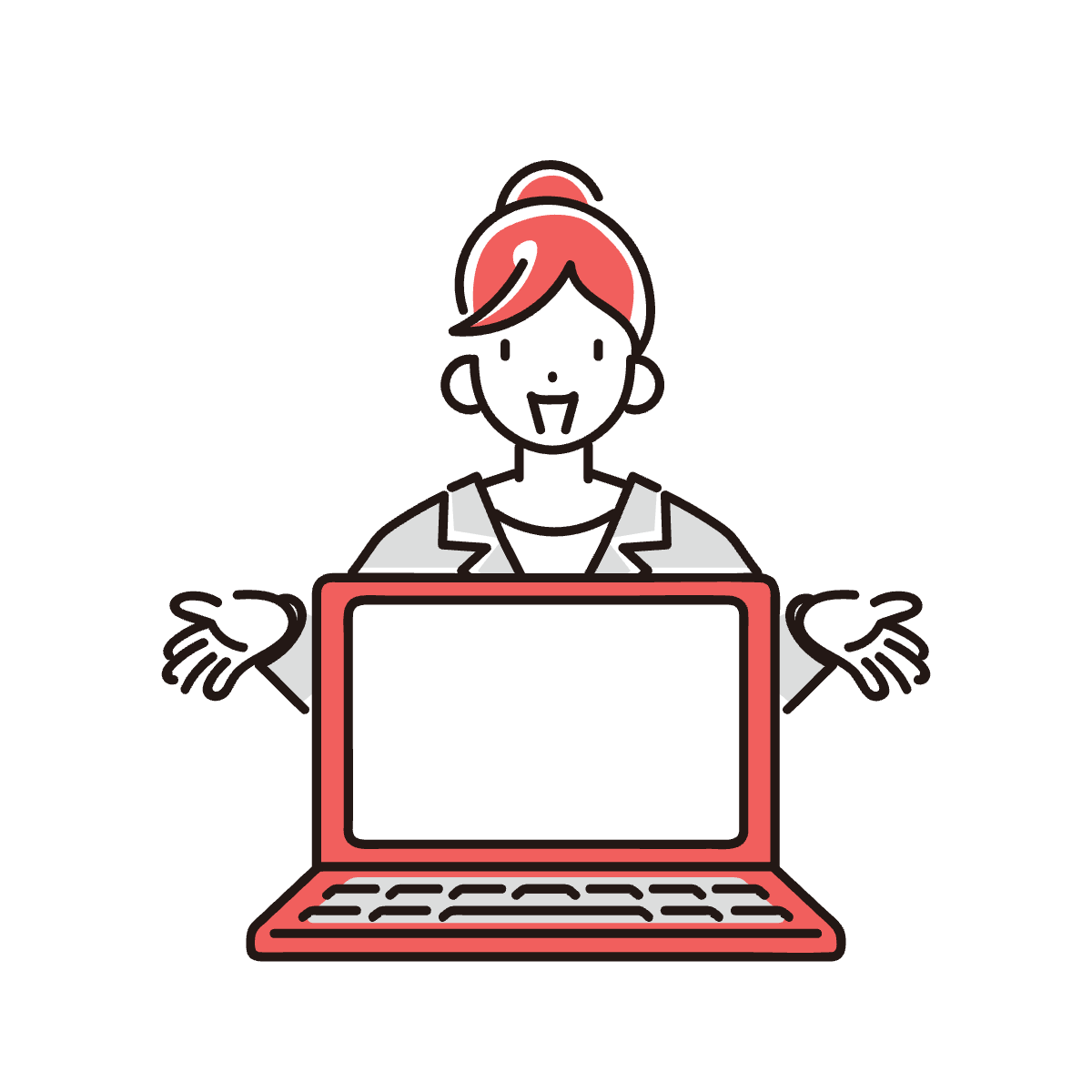
References:
🔍 Check out the demo project in github.
📮 If find any errors, please feel free to discuss and correct them: biqingsue@[google email].com.